In this tutorial, we will walk you through the process of integrating the Agora Web SDK with a Laravel 11 application. Agora provides real-time engagement APIs that enable voice, video, and interactive broadcasting. By following this guide, you will learn how to use the Agora SDK in Laravel, creating a fully functional application.
Table of Contents
What is Agora in Laravel?
Agora is a powerful SDK that enables real-time communication features such as voice and video calling. In Laravel, integrating Agora allows developers to add these capabilities to their applications, enhancing user experience and engagement.
How to Use Agora SDK?
To get started with using the Agora SDK in a Laravel application, follow the steps outlined below. Each step includes the necessary code snippets and explanations to ensure you can implement this integration smoothly.
Step 1: Set Up a New Laravel 11 Project
First, you need to set up a new Laravel project. Open your terminal and run the following command:
composer create-project --prefer-dist laravel/laravel agora-laravel-app
Navigate to the project directory:
cd agora-laravel-app
Step 2: Install Necessary Packages
You will need to install the Agora Web SDK and other dependencies. To do this, you can use npm. Run the following commands:
npm install agora-rtc-sdk-ng
npm install axios
npm install vue
Step 3: Set Up Agora Credentials
Sign up for an Agora account and create a new project. Obtain the App ID and App Certificate from the Agora dashboard. Add these credentials to your .env file:
AGORA_APP_ID=your_agora_app_id
AGORA_APP_CERTIFICATE=your_agora_app_certificate
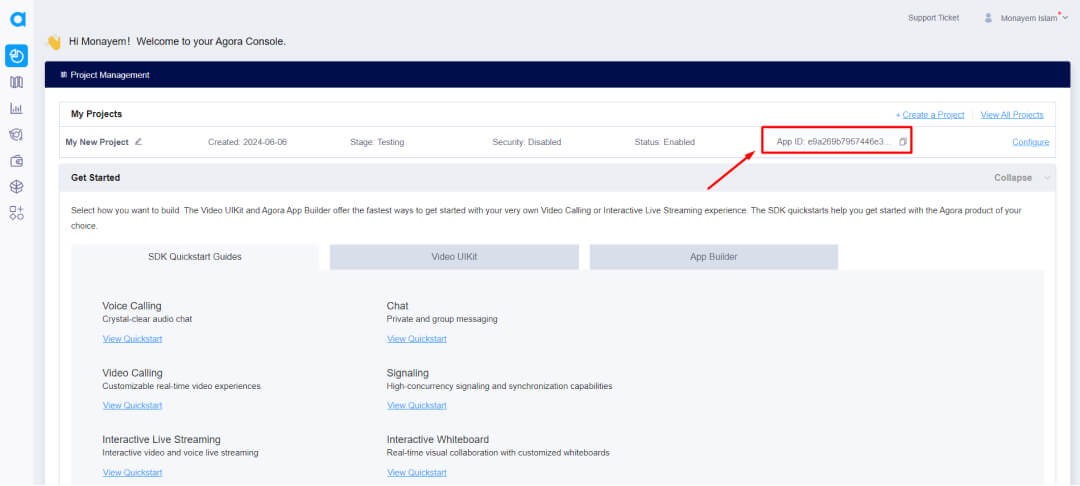
Step 4: Create Backend API for Token Generation
Agora requires a token for secure communication. Create a new controller to handle token generation. Run the following command to create a controller:
php artisan make:controller AgoraTokenController
In AgoraTokenController.php, add the following code:
channel;
$uid = 0; // User ID. A non-zero integer
$expireTimeInSeconds = 3600;
$currentTimestamp = (new \DateTime("now", new \DateTimeZone("UTC")))->getTimestamp();
$privilegeExpiredTs = $currentTimestamp + $expireTimeInSeconds;
$token = RtcTokenBuilder::buildTokenWithUid($appId, $appCertificate, $channelName, $uid, \Agora\AgoraDynamicKey\Role::ROLE_PUBLISHER, $privilegeExpiredTs);
return response()->json(['token' => $token]);
}
}
Add the following route in routes/api.php:
Route::post('/agora-token', [AgoraTokenController::class, 'generateToken']);
Step 5: Set Up Frontend for Video Call
Create a Vue component to handle the video call. In resources/js/components
, create a new file called VideoCall.vue
:
Step 6: Compile Assets
Compile your assets using the following command:
npm run dev
Step 7: Run Your Laravel Application
Start your Laravel application:
php artisan serve
Navigate to URL (such as http://localhost:8000 ) and you should see the “Start Call” button. Clicking it will initiate a video call using Agora.
Final Words - Agora Web SDK with Laravel
In this guide, we walked through the process of integrating the Agora Web SDK with a Laravel 11 application. We covered setting up a new Laravel project, installing necessary packages, generating Agora tokens, and creating a frontend component for video calls. By following these steps, you can enhance your Laravel applications with real-time communication features using Agora.
Remember to keep your Agora App ID and App Certificate secure, and always follow best practices for handling sensitive data. Happy coding!